项目 HTTP API
项目 HTTP API
项目 HTTP API 为您提供了项目级别的 API 接口,包括对项目、设备类型、设备组、设备信息、设备扩展信息、设备数据、设备通信、设备告警、用户等全面的资源访问,采用 HTTP RESTful API 风格。
准备工作
如何获得项目 API 接入地址?
在 ThingsCloud 控制台的项目中,进入任意一个设备详情页,在 连接 页面中可以找到 应用端 API 接入点,请使用 https
加密访问,确保数据安全性。
不同项目的 API 接入点地址可能不同,请您以控制台获取的地址为准。
创建 API 应用
在正式使用 API 之前,您还需要进入 ThingsCloud 控制台 > 项目应用,创建 API 类型的项目应用,获得 AppID 和 API Key,用于请求 API 时的身份验证。
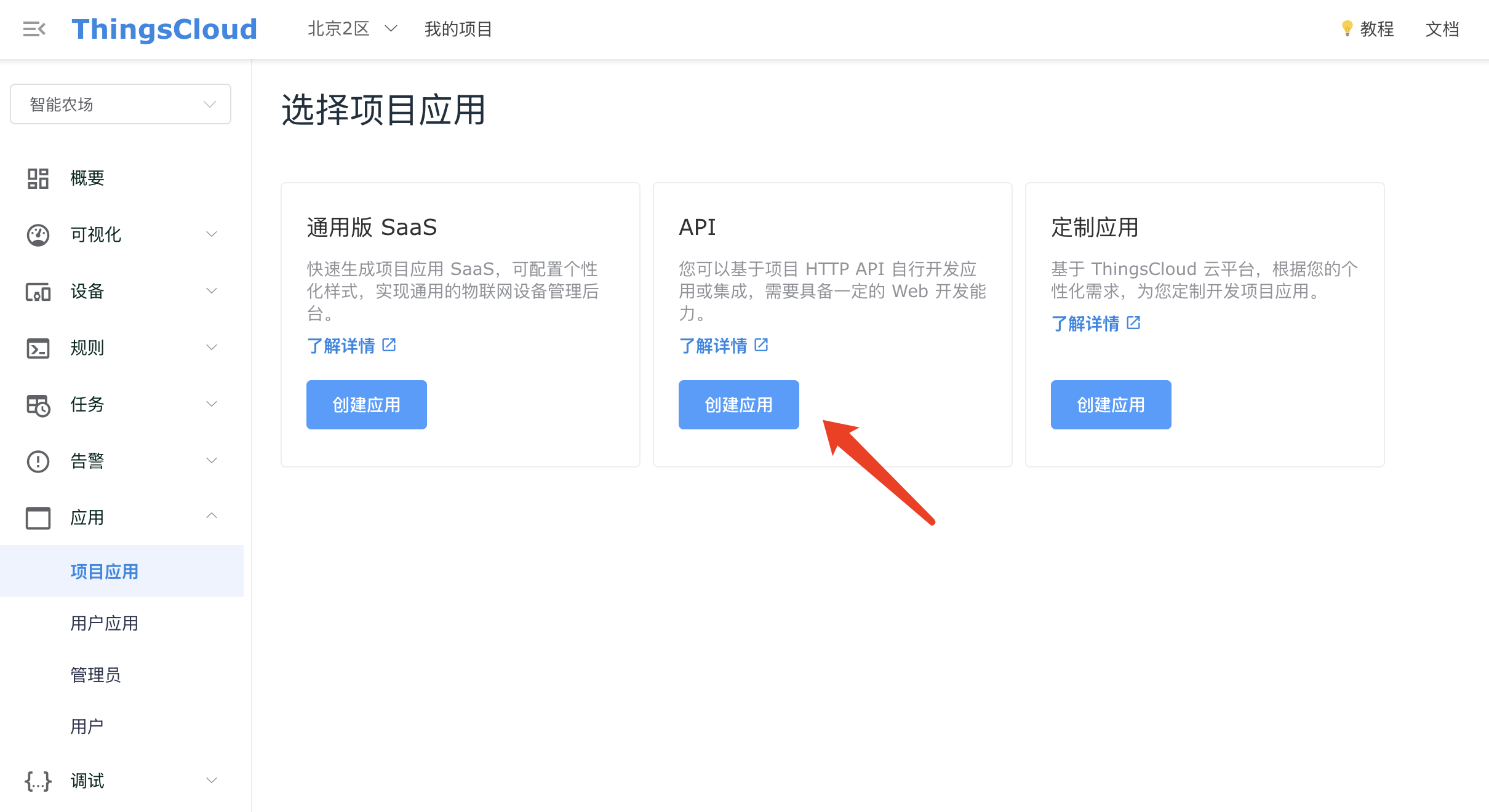
API 请求代码示例
以获取 API AccessToken 为例,以下是常用编程语言请求 API 的示例代码,仅供参考:
const axios = require('axios');
const postData = {
app_id: '<app_id>',
access_key: '<access_key>',
secret_key: '<secret_key>'
};
axios.post('https://<api-endpoint>/api/v1/access_token', postData, {
headers: {
'Content-Type': 'application/json'
}
})
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
import requests
import json
url = 'https://<api-endpoint>/api/v1/access_token'
data = {
'app_id': '<app_id>',
'access_key': '<access_key>',
'secret_key': '<secret_key>'
}
headers = {
'Content-Type': 'application/json'
}
response = requests.post(url, data=json.dumps(data), headers=headers)
print(response.text)
<?php
$api_url = 'https://<api-endpoint>/api/v1/access_token';
$data = array(
'app_id' => '<app_id>',
'access_key' => '<access_key>',
'secret_key' => '<secret_key>'
);
$payload = json_encode($data);
$ch = curl_init($api_url);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "POST");
curl_setopt($ch, CURLOPT_POSTFIELDS, $payload);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json'
));
$result = curl_exec($ch);
curl_close($ch);
echo $result;
?>
package main
import (
"bytes"
"encoding/json"
"net/http"
)
func main() {
url := "https://<api-endpoint>/api/v1/access_token"
data := map[string]string{"app_id": "<app_id>", "access_key": "<access_key>", "secret_key": "<secret_key>"}
payload, _ := json.Marshal(data)
req, _ := http.NewRequest("POST", url, bytes.NewBuffer(payload))
req.Header.Set("Content-Type", "application/json")
client := &http.Client{}
resp, _ := client.Do(req)
defer resp.Body.Close()
}
import java.net.HttpURLConnection;
import java.net.URL;
import java.io.OutputStream;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class APICallExample {
public static void main(String[] args) {
try {
// 设置 API 地址
URL url = new URL("https://<api-endpoint>/api/v1/access_token");
// 打开连接
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json");
// 设置请求正文
JSONObject jsonInput = new JSONObject();
jsonInput.put("app_id", "<app_id>");
jsonInput.put("access_key", "<access_key>");
jsonInput.put("secret_key", "<secret_key>");
String input = jsonInput.toString();
OutputStream os = conn.getOutputStream();
os.write(input.getBytes());
os.flush();
// 处理返回结果
BufferedReader br = new BufferedReader(new InputStreamReader((conn.getInputStream())));
String output;
while ((output = br.readLine()) != null) {
System.out.println(output);
}
// 关闭连接
conn.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
}
using System;
using System.IO;
using System.Net;
using System.Text;
using Newtonsoft.Json;
class APICallExample
{
static void Main(string[] args)
{
var request = (HttpWebRequest)WebRequest.Create("https://<api-endpoint>/api/v1/access_token");
request.Method = "POST";
request.ContentType = "application/json";
var payload = new
{
app_id = "<app_id>",
access_key = "<access_key>",
secret_key = "<secret_key>"
};
var jsonPayload = JsonConvert.SerializeObject(payload);
using (var streamWriter = new StreamWriter(request.GetRequestStream()))
{
streamWriter.Write(jsonPayload);
streamWriter.Flush();
streamWriter.Close();
}
var response = (HttpWebResponse)request.GetResponse();
var responseStream = response.GetResponseStream();
var streamReader = new StreamReader(responseStream);
var responseText = streamReader.ReadToEnd();
Console.WriteLine(responseText);
}
}
获取 API AccessToken
在使用下边的 API 之前,需要先调用身份验证 API,获得 AccessToken
,用于所有 API 请求中的身份标识。
提示
请注意,这里的项目 HTTP API 中的 AccessToken
不是 设备证书中的 AccessToken
,它们只是名字相同,没有任何关系,请勿混用。
身份验证 API 支持以下两种方式:
服务器获取 AccessToken
通过服务器发起请求,获取 AccessToken
。这种方式,API 密钥保存在服务器,可有效防止泄露。
AccessToken
获取后的有效期为 1 天,过期后请重新获取。
Request Syntax
POST /api/v1/access_token HTTP/1.1
Content-Type: application/json
{
"app_id": "string",
"access_key": "string",
"secret_key": "string"
}
Request Body
Field | Type | Required | Description |
---|---|---|---|
app_id | string | Yes | 在控制台项目应用中获取 |
access_key | string | Yes | 在控制台项目应用中获取 |
secret_key | string | Yes | 在控制台项目应用中获取 |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"access_token": "string",
"expires_in": 86400
}
}
浏览器获取 AccessToken
通过管理账号和密码,获取 AccessToken
。该方式适用于在浏览器网页中由浏览者输入账号和密码,通过浏览器发起请求,并将成功获取的 AccessToken
保存到 Cookies 中。
如何创建管理员账号,请浏览 管理员账号。
AccessToken
获取后的有效期为 7 天,过期后请重新获取。
Request Syntax
POST /api/v1/admin_login HTTP/1.1
Content-Type: application/json
{
"app_id": "string",
"account": "string",
"password": "string"
}
Request Body
Field | Type | Required | Description |
---|---|---|---|
app_id | string | Yes | 项目应用 AppID,在控制台项目应用中获得 |
account | string | Yes | 管理员登录名 |
password | string | Yes | 管理员密码 |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"access_token": "string",
"expires_in": 604800
}
}
项目信息
读取项目信息
读取当前项目的信息。
Request Syntax
GET /api/v1/project HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"id": "string",
"name": "string",
"descr": "string",
"tags": {}
}
}
读取设备状态统计
读取项目中所有设备基本状态的统计。
Request Syntax
GET /api/v1/devices_stat HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Query Parameters
Parameter | Required | Description |
---|---|---|
groups | No | 设备组ID,可查询指定设备组下所有设备的状态统计。支持多个设备组ID,以逗号分隔。 |
include_sub_groups | No | 若指定设备组ID,可设置是否包含子设备组。1 表示包含子设备组,默认为不包含。 |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"device_total": number,
"online_device_total": number,
"offline_device_total": number,
"active_device_total": number,
"inactive_device_total": number,
"alarm_device_total": number
}
}
Field | Type | Description |
---|---|---|
device_total | number | 总设备数 |
online_device_total | number | 在线设备数 |
offline_device_total | number | 离线设备数 |
active_device_total | number | 活跃设备数 |
inactive_device_total | number | 非活跃设备数 |
alarm_device_total | number | 告警设备数 |
读取设备告警状态统计
读取项目中所有设备告警状态的统计。
Request Syntax
GET /api/v1/devices_alarm_stat HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Query Parameters
Parameter | Required | Description |
---|---|---|
groups | No | 设备组ID,可查询指定设备组下所有设备的状态统计。支持多个设备组ID,以逗号分隔。 |
include_sub_groups | No | 若指定设备组ID,可设置是否包含子设备组。1 表示包含子设备组,默认为不包含。 |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"device_total": number,
"alarm_1_total": number,
"alarm_2_total": number,
"alarm_3_total": number,
"normal_total": number,
}
}
Field | Type | Description |
---|---|---|
device_total | number | 总设备数 |
alarm_1_total | number | 普通告警设备数 |
alarm_2_total | number | 严重告警设备数 |
alarm_3_total | number | 紧急告警设备数 |
normal_total | number | 正常设备数 |
设备类型
读取设备类型列表
读取所有设备类型列表。
Request Syntax
GET /api/v1/device_types HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"items": [
{
"id": "string",
"name": "string",
"descr": "string",
"tags": {
"key1": "string",
"key2": "string"
},
"create_time": number,
"device_count": number,
"conn_protocol": "string",
"conn_type": "string"
},
...
]
}
}
Field | Type | Description |
---|---|---|
id | string | ID |
name | string | 名称 |
descr | string | 描述 |
tags | object | 标签 |
create_time | number | 创建时间 |
device_count | number | 关联的设备数量 |
conn_protocol | string | 设备通信方式 |
conn_type | string | 设备接入方式 |
读取设备类型下的设备列表
请见 读取设备列表
读取设备类型扩展信息定义
读取指定设备类型的扩展信息定义。
Request Syntax
GET /api/v1/device_type/<device_type_id>/extend_infos HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Path Parameters
Parameter | Description |
---|---|
device_type_id | 设备类型 ID |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"items": [
{
"id": "string",
"info_type": "string",
"name": "string",
"descr": "string",
"enabled": boolean,
"options": {},
"create_time": number,
"update_time": number,
"is_standard": boolean
},
]
}
}
设备组
读取设备组树形列表
读取所有设备组的树形列表,包含子组。
Request Syntax
GET /api/v1/device_groups_tree HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"items": [
{
"id": "string",
"name": "string",
"descr": "string",
"tags": {
"key1": "string",
"key2": "string"
},
"create_time": number,
"device_count": 10,
"children": [
{
"id": "string",
"name": "string",
"descr": "string",
"tags": {
"key1": "string",
"key2": "string"
},
}
...
]
},
...
]
}
}
Field | Type | Description |
---|---|---|
id | string | 设备组ID |
name | string | 名称 |
descr | string | 描述 |
tags | object | 标签 |
create_time | number | 创建时间,Unix 时间戳(毫秒) |
device_count | number | 分组中的设备数 |
children | array | 子组数组 |
读取设备组下的设备列表
请见 读取设备列表
设备管理
读取设备列表
读取所有设备列表。
Request Syntax
GET /api/v1/devices HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Query Parameters
Parameter | Required | Description |
---|---|---|
page | No | 页码,默认为1 |
type | No | 指定设备类型ID |
groups | No | 指定设备组ID,支持多个设备组ID,用逗号分隔。groups= 空值表示查询未分组的设备。 |
include_sub_groups | No | 若指定设备组ID,可设置是否包含子设备组。1 表示包含子设备组,默认为不包含。 |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"items": [
{
"id": "string",
"name": "string",
"descr": "string",
"device_key": "string",
"tags": {
"key1": "string",
"key2": "string"
},
"create_time": number,
"alarm": {},
"active": {},
"online": boolean,
"type": "string",
"type_info": {
"id": "string",
"name": "string",
"conn_type": "string",
"conn_protocol": "string"
},
"groups": [
"string",
...
],
"device_code": "string"
},
...
],
"total": number,
"page_total": number,
}
}
Field | Type | Description |
---|---|---|
items | array | 设备数组 |
total | number | 设备总数 |
page_total | number | 总页数 |
items[]
Object:
Field | Type | Description |
---|---|---|
id | string | 设备ID |
name | string | 设备名称 |
descr | string | 设备描述 |
device_key | string | 设备唯一标识 DeviceKey |
tags | object | 标签 |
create_time | number | 设备创建时间 |
alarm | object | 设备告警状态 |
active | object | 设备各类消息最新时间 |
online | boolean | 设备在线状态 |
type | string | 设备类型ID |
type_info | object | 设备类型信息 |
groups | array | 设备组ID |
device_code | string | 设备码 |
读取设备信息
读取指定设备的信息。
Request Syntax
GET /api/v1/device/<device_id> HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Path Parameters:
Parameter | Description |
---|---|
device_id | 设备 ID |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"id": "string",
"name": "string",
"descr": "string",
"device_key": "string",
"tags": {
"key1": "string",
"key2": "string"
},
"create_time": number,
"alarm": {},
"active": {},
"online": boolean,
"type": "string",
"type_info": {
"id": "string",
"name": "string",
"conn_type": "string",
"conn_protocol": "string"
},
"groups": [
"string",
"string"
],
"device_code": "string",
"view_token": "string"
}
}
Field | Type | Description |
---|---|---|
id | string | 设备ID |
name | string | 设备名称 |
descr | string | 设备描述 |
device_key | string | 设备唯一标识 DeviceKey |
tags | object | 标签 |
create_time | number | 设备创建时间 |
alarm | object | 设备告警状态 |
active | object | 设备各类消息最新时间 |
online | boolean | 设备在线状态 |
type | string | 设备类型ID |
type_info | object | 设备类型信息 |
groups | array | 设备组ID |
device_code | string | 设备码 |
view_token | string | 设备 DeviceViewToken ,用在 MQTT 应用端订阅 |
读取设备证书
读取指定设备的连接证书。
Request Syntax
GET /api/v1/device/<device_id>/certificate HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"id": "string",
"name": "string",
"access_token": "string",
"project_key": "string"
}
}
读取设备用户列表
读取指定设备关联的所有用户。
Request Syntax
GET /api/v1/device/<device_id>/customers HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Response Syntax
参考 读取用户列表
修改设备基本信息
修改指定设备的基本信息。
Request Syntax
PUT /api/v1/device/<device_id>/info HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"name": "string",
"descr": "string",
"device_key": "string"
}
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Request Body
Field | Type | Required | Description |
---|---|---|---|
name | string | No | 设备名称 |
descr | string | No | 设备描述 |
device_key | string | No | 设备唯一标识 DeviceKey |
必须包含至少一个字段。
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {}
}
修改设备标签
修改设备的标签。
Request Syntax
PUT /api/v1/device/<device_id>/tags HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"tags": {
"key1": "string",
"key2": "string",
...
}
}
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Request Body
Field | Type | Required | Description |
---|---|---|---|
tags | object | Yes | 要更新或增加的标签集合 |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {}
}
移除设备标签
移除设备的标签。
Request Syntax
POST /api/v1/device/<device_id>/tags_remove HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"keys": [
"string",
"string",
...
]
}
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Request Body
Field | Type | Required | Description |
---|---|---|---|
keys | array | Yes | 要移除的标签 Key 数组,可同时移除一个或多个标签。 |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {}
}
修改设备分组
修改设备所在的分组。
Request Syntax
PUT /api/v1/device/<device_id>/groups HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"groups": [
"string",
"string",
...
]
}
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Request Body
Field | Type | Required | Description |
---|---|---|---|
groups | array | Yes | 要更新的分组 ID 数组。空数组表示设备不属于任何分组。 |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {}
}
移除设备
移除指定设备。
Request Syntax
DELETE /api/v1/device/<device_id> HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {}
}
创建设备
创建新设备。
Request Syntax
POST /api/v1/device HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"name": "string",
"descr": "string",
"type": "string",
"tags": {},
"device_key": "string"
}
Request Body
Field | Type | Required | Description |
---|---|---|---|
name | string | Yes | 设备名称 |
descr | string | No | 设备描述 |
type | string | No | 设备类型 ID |
tags | object | No | 设备标签 |
device_key | string | No | 设备唯一标识 DeviceKey |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"id": "string",
"name": "string"
}
}
设备扩展信息
读取设备扩展信息
读取指定设备的所有扩展信息。
Request Syntax
GET /api/v1/device/<device_id>/extend_infos HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"items": [
{
"id": "string",
"info_type": "string",
"name": "string",
"descr": "string",
"value": "string"|boolean|number|object,
"options": {},
"create_time": number,
"update_time": number,
"is_standard": boolean
},
]
}
}
修改设备标准扩展信息
修改指定设备的标准扩展信息。
Request Syntax
POST /api/v1/device/<device_id>/standard_infos HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"info_type": "string",
"value": {
"key": "string"|boolean|number,
}
}
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Request Body
Field | Type | Required | Description |
---|---|---|---|
info_type | string | Yes | 扩展信息类型 |
value | object | Yes | 扩展信息取值 |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"<info_type>": {
"key": "string"|boolean|number
}
}
}
返回的 info
中会显示更新成功的扩展信息。
修改设备自定义扩展信息
修改指定设备的标准扩展信息。
Request Syntax
POST /api/v1/device/<device_id>/extend_infos HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"infos": {
"<info-id-1>": "string"|boolean|number,
"<info-id-2>": "string"|boolean|number,
...
}
}
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Request Body
Field | Type | Required | Description |
---|---|---|---|
<info-id> | string | Yes | 自定义扩展信息的 ID,可通过设备类型扩展信息定义接口获得。 |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"<info-id-1>": "string"|boolean|number,
"<info-id-2>": "string"|boolean|number,
...
}
}
返回的 info
中会显示更新成功的扩展信息。
设备数据
读取设备属性列表
读取设备的所有属性列表,包含相关的属性定义。
Request Syntax
GET /api/v1/device/<device_id>/attributes HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": [
{
"identifier": "string",
"value": any,
"attr_type": "string",
"data_type": "string",
"ts": number,
"model": {
"name": "string",
"attr_type": "string",
"data_type": "string",
"data_options": {
}
}
},
]
}
Field | Type | Description |
---|---|---|
identifier | string | 属性标识符 |
value | any | 属性值 |
attr_type | string | 属性类型。report :属性上报, push :云端下发,cloud :云端私有 |
data_type | string | 数据类型 |
ts | string | 最后更新时间,Unix 时间戳(毫秒)格式 |
model | object | 属性定义信息。若该属性无属性定义,则无该字段。 |
model
Object:
Field | Type | Description |
---|---|---|
name | string | 属性名称 |
attr_type | string | 属性类型。属性类型。report :属性上报, push :云端下发, share :设备云端共享,cloud :云端私有 |
data_type | string | 属性定义中的数据类型 |
data_options | object | 属性定义选项 |
读取设备当前属性值集合
读取设备当前属性的集合,只包含属性标识符和属性值。
Request Syntax
GET /api/v1/device/<device_id>/attributes_raw HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Query Parameters
Parameter | Required | Description |
---|---|---|
keys | No | 可指定属性标识符,多个用逗号风格。例如:temperature,humidity,co2 。不指定默认返回所有属性。 |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"temperature": 24.1,
"humidity": 56.8,
"relay1": true,
"relay2": false,
"relay3": false
}
}
读取设备当前属性元信息
读取设备当前属性的元信息。
Request Syntax
GET /api/v1/device/<device_id>/attributes_meta HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Query Parameters
Parameter | Required | Description |
---|---|---|
keys | No | 可指定属性标识符,多个用逗号风格。例如:temperature,humidity,co2 。不指定默认返回所有属性。 |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"switch1": {
"type": "string",
"data_type": "string",
"ts": number
},
"temperature": {
"type": "string",
"data_type": "string",
"ts": number
}
}
}
Response Syntax
元信息
Field | Type | Description |
---|---|---|
type | string | 属性类型 |
data_type | string | 数据类型 |
ts | number | 最后更新时间,Unix 时间戳(毫秒)格式 |
读取设备属性历史数据
读取设备指定属性的历史数据。
Request Syntax
GET /api/v1/device/<device_id>/attribute/<identifier>/series HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
identifier | 属性标识符 |
Query Parameters
Parameter | Required | Description |
---|---|---|
page | No | 页码,默认为1 |
page_records | No | 每页记录数,数值范围为 1-500,默认为 20 |
start_time | No | 指定历史数据的开始时间,Unix 时间戳(毫秒)格式 |
end_time | No | 指定历史数据的结束时间,Unix 时间戳(毫秒)格式 |
start_time
和 end_time
同时指定时,将查询指定时间范围的历史数据,否则默认查询从当前时间往前 30 天的历史数据。
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": [
{
"timestamp": "string",
"ts": number,
"name": "string",
"value": any,
"type": "string"
},
...
]
}
Field | Type | Description |
---|---|---|
timestamp | string | 数据更新时间,ISO 8601 格式 |
ts | string | 数据更新时间,Unix 时间戳(毫秒)格式 |
name | string | 属性标识符 |
value | string | 属性值 |
type | string | 属性类型。report :属性上报, push :云端下发,cloud :云端私有 |
读取设备属性聚合统计数据
读取设备指定属性的聚合统计数据,例如:计算特定时间范围内的最大值、最小值、平均值、总和等。
Request Syntax
GET /api/v1/device/<device_id>/attribute/<identifier>/aggregate HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
identifier | 属性标识符,仅支持数值类型的属性。 |
Query Parameters
Parameter | Required | Description |
---|---|---|
time_range | No | 数据时间范围,默认为last_1h (最近1小时)。取值包括: last_5m (最近5分钟)、last_15m (最近15分钟)、last_30m (最近30分钟)、last_1h (最近1小时)、last_3h (最近3小时)、last_6h (最近6小时)、last_12h (最近12小时)、last_1d (最近1天)、last_3d (最近3天)、last_7d (最近7天)、last_15d (最近15天)、last_30d (最近30天)、last_60d (最近60天)、last_90d (最近90天)、last_6mo (最近6个月)、last_1y (最近1年)、today (今天)、yday (昨天)、this_week (本周)、prev_week (上周)、this_month (本月)、prev_month (上个月)、this_year (今年)、custom (自定义时间范围) |
start_time | No | 当time_range 等于custom 时,指定数据的开始时间,Unix 时间戳(毫秒)格式 |
end_time | No | 当time_range 等于custom 时,指定数据的结束时间,Unix 时间戳(毫秒)格式 |
window | No | 聚合计算时间,默认为1h (1小时)。取值包括: 1m (1分钟)、2m (2分钟)、5m (5分钟)、10m (10分钟)、30m (30分钟)、1h (1小时)、3h (3小时)、6h (6小时)、1d (1天)、7d (7天)、1mo (1个月) |
function | No | 聚合计算方法,默认为avg 。取值包括: avg (平均值)、max (最大值)、min (最小值)、median (中位数)、first (首位数)、last (末尾数)、count (次数统计)、spread (首尾差值)、sum (求和) |
例如:
- 读取最近30天的每小时最大值,请求参数如下:
time_range=last_30d&window=1h&function=max
- 读取 2023-12-01 至 2024-03-01 之间每天的平均值,请求参数如下:
time_range=custom&start_time=1701360000000&end_time=1709222400000&window=1d&function=avg
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"time_range": {
"start": number,
"end": number
},
"window_duration": number,
"function": "string",
"data": [
{
"timestamp": "string",
"ts": number,
"value": number
}
]
}
}
Field | Type | Description |
---|---|---|
time_range | object | 实际读取的数据时间范围 |
window_duration | number | 聚合计算时间长度(秒数) |
function | string | 聚合计算方法 |
data | array | 聚合数据 |
data[]
Object:
Field | Type | Description |
---|---|---|
timestamp | string | 数据时间,ISO 8601 格式 |
ts | string | 数据时间,Unix 时间戳(毫秒)格式 |
value | string | 数据值 |
移除设备属性
移除设备的指定属性,可同时移除一个或多个属性。
Request Syntax
POST /api/v1/device/<device_id>/attributes_remove HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"keys": [
"string",
"string",
...
]
}
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Request Body
Field | Type | Required | Description |
---|---|---|---|
keys | array | Yes | 要移除的属性标识符数组,可包含多个属性标识符,例如:["temperature","humitidy"] |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {}
}
设备通信
下发属性到设备
向设备下发属性数据。
Request Syntax
POST /api/v1/control/device/<device_id>/attributes HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"key1": value,
"key2": value,
...
}
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {}
}
更新云端属性
更新设备的云端属性。
Request Syntax
PUT /api/v1/control/device/<device_id>/attributes HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"key1": value,
"key2": value,
...
}
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {}
}
下发命令到设备
向设备下发命令消息。
Request Syntax
POST /api/v1/control/device/<device_id>/command HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"method": "string",
"params": {
"key1": value1,
"key2": value2,
...
},
"id": number
}
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Request Body
下发命令消息的详细介绍,请浏览 设备接收云端下发命令
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {}
}
下发自定义数据流到设备
向设备下发自定义数据流消息。
Request Syntax
POST /api/v1/control/device/<device_id>/data/<identifier>/set?push_mode=<push_mode> HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"type": "string",
"msg": <Message Body>,
}
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
identifier | 自定义数据流标识符,例如:stream |
Query Parameters
Parameter | Required | Description |
---|---|---|
push_mode | Yes | 自定义数据流下发通道,可填 mqtt 或 tcp |
Request Body
根据自定义数据流设置的不同消息类型,API 请求正文的 <Message Body>
具体格式和用法举例如下:
- 下发
HEX
消息格式
{
"type": "hex",
"msg": "01 03 01 FF 00 0C 74 03"
}
- 下发
Plaintext
消息格式
{
"type": "text",
"msg": "t:45,u=31"
}
- 下发
JSON
消息格式
{
"type": "json",
"msg": {
"command": "openLock",
"led": "ON",
"stat": "011001"
}
}
关于自定义数据流的基本概念,请浏览 自定义数据流
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {}
}
设备告警
读取所有告警消息
读取项目中的所有告警消息历史。
Request Syntax
GET /api/v1/alarm_logs HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"items": [
"id": "string",
"device_id": "string",
"alarm_status": number,
"alarm_level": number,
"alarm_info": {},
"create_time": number,
"notify_logs": [],
"device": {
"id": "string",
"name": "device2"
}
]
}
}
读取设备告警消息
读取指定设备的告警消息历史。
Request Syntax
GET /api/v1/device/<device_id>/alarm_logs HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Path Parameters
Parameter | Description |
---|---|
device_id | 设备 ID |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"items": [
"id": "string",
"device_id": "string",
"alarm_status": number,
"alarm_level": number,
"alarm_info": {},
"create_time": number,
"notify_logs": [],
"device": {
"id": "string",
"name": "device2"
}
]
}
}
用户管理
读取用户列表
读取项目中的所有用户列表。
Request Syntax
GET /api/v1/customers HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"items": [
{
"id": "string",
"account": "string",
"name": "string",
"descr": "string",
"role_id": "string",
"email": "string",
"mobile": "string",
"tags": {},
"locale": "string",
"create_time": number,
"count": {
"device_count": number
}
}
]
}
}
读取用户角色列表
读取项目中的所有用户角色列表。
Request Syntax
GET /api/v1/customer_roles HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"items": [
{
"id": "string",
"name": "string",
"descr": "string",
"allow_operate_device": boolean,
"allow_alarm_msg_maintain": boolean,
"allow_add_device": boolean,
"create_time": number,
"customer_count": number
}
]
}
}
读取用户信息
读取指定用户的信息。
Request Syntax
GET /api/v1/customer/<customer_id> HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Path Parameters
Parameter | Description |
---|---|
customer_id | 用户 ID |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"id": "string",
"account": "string",
"name": "string",
"descr": "string",
"email": "string",
"mobile": "string",
"tags": {},
"locale": "string",
"create_time": number,
"count": {
"device_count": number
}
}
}
读取用户设备列表
读取用户关联的设备列表。
Request Syntax
GET /api/v1/customer/<customer_id>/devices HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Path Parameters
Parameter | Description |
---|---|
customer_id | 用户 ID |
Query Parameters
page
:可选,页码,默认为 1
Response Syntax
参考 读取设备列表
创建用户
为项目创建新用户。
Request Syntax
POST /api/v1/customer HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"name": "string",
"account": "string",
"password": "string",
"descr": "string",
"email": "string",
"mobile": "string",
"tags": {}
}
Request Body
Field | Type | Required | Description |
---|---|---|---|
name | string | Yes | 用户名称 |
account | string | Yes | 用户登录名 |
password | string | Yes | 用户登录密码 |
descr | string | No | 用户备注信息 |
email | string | No | 用户邮箱 |
mobile | string | No | 用户手机号 |
tags | object | No | 用户标签键值对 |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"id": "string",
"name": "string"
}
}
修改用户信息
修改指定用户的基本信息。
Request Syntax
PUT /api/v1/customer/<customer_id>/info HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"name": "string",
"descr": "string",
"email": "string",
"mobile": "string"
}
Path Parameters
Parameter | Description |
---|---|
customer_id | 用户 ID |
Request Body
Field | Type | Required | Description |
---|---|---|---|
name | string | No | 用户名称 |
descr | string | No | 用户备注信息 |
email | string | No | 用户邮箱 |
mobile | string | No | 用户手机号 |
以上字段至少填写一个,可修改一个或多个字段。
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {}
}
修改用户密码
修改指定用户的登录信息。
Request Syntax
PUT /api/v1/customer/<customer_id>/pwd HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"password": "string",
}
Path Parameters
Parameter | Description |
---|---|
customer_id | 用户 ID |
Request Body
Field | Type | Required | Description |
---|---|---|---|
password | string | Yes | 用户登录密码 |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {}
}
修改用户标签
修改指定用户的标签。
Request Syntax
PUT /api/v1/customer/<customer_id>/tags HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"tags": {
"key1": "string",
"key2": "string",
...
}
}
Path Parameters
Parameter | Description |
---|---|
customer_id | 用户 ID |
Request Body
Field | Type | Required | Description |
---|---|---|---|
tags | object | Yes | 要更新或增加的标签集合 |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {}
}
移除用户
移除指定的用户账号。
Request Syntax
DELETE /api/v1/customer/<customer_id> HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Path Parameters
Parameter | Description |
---|---|
customer_id | 用户 ID |
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {}
}
用户登录验证
验证用户账号和密码是否正确。
Request Syntax
POST /api/v1/customer_auth HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
{
"account": "string",
"password": "string",
}
Request Body
Field | Type | Required | Description |
---|---|---|---|
account | string | Yes | 用户登录名 |
password | string | Yes | 用户登录密码 |
Response Syntax
- 验证成功
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"id": "string",
"name": "string"
}
}
- 验证失败
HTTP/1.1 200 OK
Content-type: application/json
{
"result": false,
"errcode": number,
"message": "string"
}
管理员管理
读取当前登录的管理员信息
读取当前登录的管理员信息,仅在通过管理员账号获取 AccessToken 时可用。
Request Syntax
GET /api/v1/admin HTTP/1.1
Content-Type: application/json
Authorization: Bearer <AccessToken>
Response Syntax
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
"id": "string",
"account": "string",
"name": "string",
"email": "string",
"tags": {}
}
}
全局定义
Response Syntax
请求功能处理成功
HTTP/1.1 200 OK
Content-type: application/json
{
"result": true,
"info": {
...
}
}
请求功能处理失败
HTTP/1.1 200 OK
Content-type: application/json
{
"result": false,
"errcode": number,
"message": "string",
"errors": [],
}
请求资源错误
当请求的 API 资源路径不存在时,返回如下:
HTTP/1.1 404 Not Found
Content-type: application/json
{
"message": "Request invalid"
}
请求 JSON 格式错误
当请求正文的 JSON 格式错误时,返回如下:
HTTP/1.1 404 Not Found
Content-type: application/json
{
"result": false,
"message": "Invalid JSON message",
"errcode": 400
}
时间格式
在 API 响应数据中,时间通常采用以下两种格式:
ISO 8601
示例:"2024-07-11T02:51:00.069Z"
这是一个字符串格式的时间戳,表示协调世界时 (UTC) 的时间。ISO 8601 格式的时间戳非常适合人类阅读和处理,尤其是在跨系统和跨语言的通信中。具体格式如下:
2024-07-11
表示日期 (年-月-日)。T
是日期和时间的分隔符。02:51:00.069
表示时间 (时:分:秒.毫秒)。Z
表示时间是以 UTC 时间表示。
适用于日志、报告、用户界面等需要展示时间的地方。
可以根据需要进行本地化处理或格式化显示,以下是 python
中的处理示例:
from datetime import datetime
iso_timestamp = "2024-07-11T02:51:00.069Z"
dt_object = datetime.fromisoformat(iso_timestamp.replace("Z", "+00:00"))
print(dt_object) # 输出: 2024-07-11 02:51:00.069000+00:00
Unix 时间戳 (毫秒)
示例:1720666260069
- 这是一个整数格式的时间戳,表示从1970年1月1日00:00:00 UTC开始经过的毫秒数。Unix 时间戳非常适合计算机内部使用,因为它简洁、易于计算时间差和进行时间比较。这个格式特别适合用于数据存储和处理时的时间标记。
- 适用于需要进行时间计算、排序、存储等操作的地方。
可以将其转换为人类可读的格式。以下是 python
中的处理示例:
import datetime
unix_timestamp = 1720666260069 / 1000.0 # 转换为秒
dt_object = datetime.datetime.utcfromtimestamp(unix_timestamp)
print(dt_object) # 输出: 2024-07-11 02:51:00.069000
API 限速规则
什么是限速?
为了确保所有用户都能获得高质量和可靠的服务,以上 HTTP API 采用动态限速规则。这些限速措施旨在防止滥用 API,并保护系统的稳定性以及提升系统安全风险。
- 保障稳定性:通过限制每个用户在一定时间内的请求次数和频率,确保服务器能够稳定地处理所有用户的请求。
- 防止滥用:防止个别用户或自动脚本过度使用资源,影响其他用户的正常使用。
- 提升安全性:保护系统和数据的安全。
如何应对限速?
当 HTTP 请求频率达到动态限速标准时,将收到 HTTP 状态码 429 Too Many Requests
。为了帮助您管理和调整请求频率,我们在响应头中提供了速率限制的相关信息。您可以通过以下 HTTP 头来查看当前的限速状态:
X-RateLimit-Limit
:当前时间窗口内允许的最大请求次数。X-RateLimit-Remaining
:当前时间窗口内剩余的可用请求次数。X-RateLimit-Reset
:当前时间窗口结束的时间(以Unix时间戳形式表示)。Retry-After
:当请求超过限制时,表示需要等待多少秒才能进行下一次请求。
这些信息将帮助您了解自己的请求状态,合理安排请求频率,从而避免触发限速。
请注意,具体的限速规则是动态调整的,以确保系统的稳定性和安全性。
感谢您的理解和配合。如果您有任何疑问或需要进一步的帮助,请随时联系我们的支持团队。